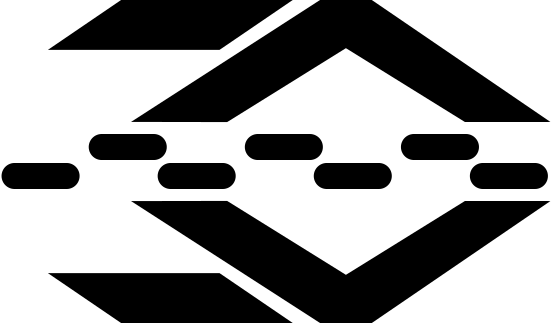
Concur
Build interactive data analysis and visualization GUIs in Python.
Concur is a library for rapidly developing user interfaces. Responsive interfaces can be written with just a few lines of code.
Build interactive data analysis and visualization GUIs in Python.
Concur is a library for rapidly developing user interfaces. Responsive interfaces can be written with just a few lines of code.
import concur as c
c.main(c.text("Hello, world!"), "Hello World", 400, 288)
import concur as c
def counter():
count = 5
while True:
tag, _ = yield from c.orr([
c.text(f"Count: {count}"),
c.button("Increment"),
c.button("Decrement"),
])
if tag == "Increment":
count += 1
elif tag == "Decrement":
count -= 1
yield
c.main(counter(), "Counter", 400, 288)
import concur as c
from PIL import Image
def app():
im = c.Image(Image.open("examples/lenna.png"))
while True:
_, im = yield from c.image("Image", im)
yield
c.main(app(), "Image", 400, 288)
import concur as c
import numpy as np
def line(tf):
np.random.seed(0)
x = np.linspace(-2, 2, 10000)
y = np.cumsum(np.random.rand(len(x)) - 0.5) / 100
return c.draw.polyline(np.stack([x, y]).T, 'blue', tf=tf)
def plot():
frame = c.Frame((-1, 1), (1, -1))
while True:
_, frame = yield from c.frame("Frame", frame, content_gen=line)
yield
c.main(plot(), "Plot", 400, 288)
import concur as c
import numpy as np
from time import monotonic as t
def graph(tf):
while True:
ts = np.linspace(t(), t() + 1/2, 100)
pts = np.stack([np.sin(ts * 4), np.cos(ts * 5)]).T
yield from c.orr([
c.draw.polyline(pts, 'blue', tf=tf),
c.event(None), # refresh every frame
])
yield
def app():
view = c.Frame((-1.5, 1.5), (1.5, -1.5))
while True:
_, view = yield from c.frame("Frame", view, content_gen=graph)
yield
if __name__ == "__main__":
c.main(app(), "Plot", 400, 288)
You need to have:
pip install concur
For more detailed instructions, see the GitHub repository.